For the upcoming Icehouse Ventures investor portal we chose to build the application using Laravel, which is a php framework. Frameworks like Laravel speed up the development process by providing a lot of the scaffolding that you need for a modern web app.
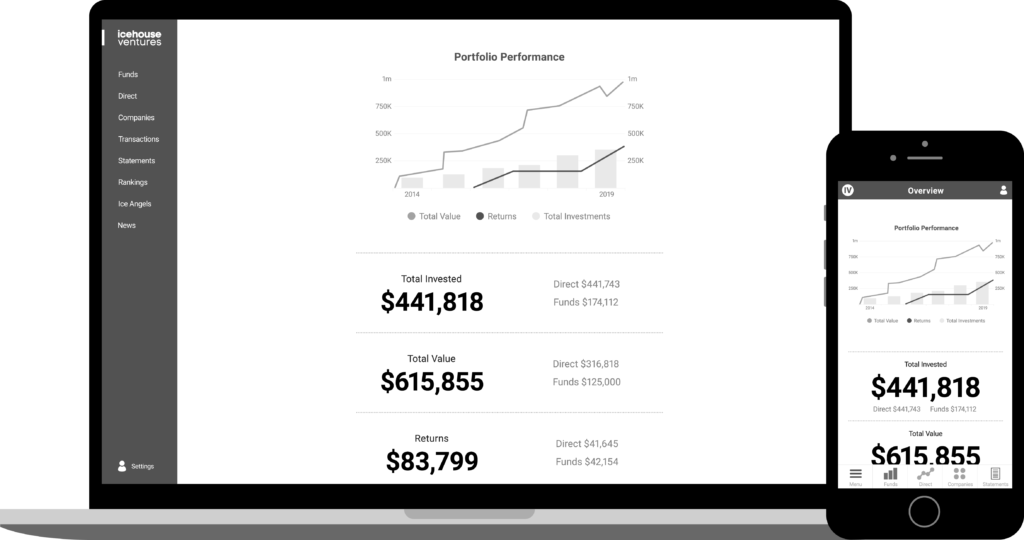
We started off looking at using off-the-shelf tools such as venture capital fund management software and various online angel network platforms. But we couldn’t find any tools that would allow us to provide combined reports that showed a consolidated view of both direct angel investments into a company and indirect fund investments into the same company through a fund the investor was a part of. Investors told us that this was a mission-critical feature so they could evaluate their effective exposure to particular startup companies in their portfolio.
We also evaluated a range of other low-code and no-code solutions such as Salesforce and various template-based databases and website builders. These were ok, but couldn’t handle the depth of complexity investors wanted such as calculating IRR over long time periods, exporting PDF statements and ranking portfolio performance against other investors.
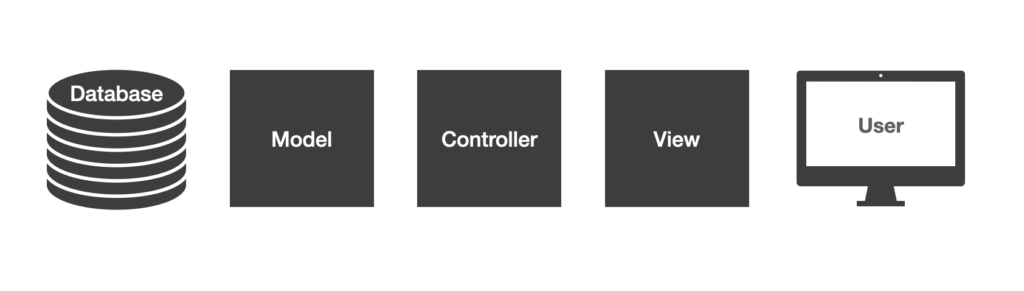
In the end, we chose php and in particular Laravel because of the robust stability of a MySQL database and the power of the Model-View-Controller approach to the code structure. MVC is a common pattern used in enterprise architecture to build scalable and stable software systems, the key elements are:
- Model – A data model that manages your database and represents data as objects that can have relationships with other objects. For example, we have a model for Users and a model for Companies in the investor portal.
- Controller – Traffic controllers that manage how data moves around your application. Controllers fetch data from the Models and send it to the Views. In Laravel, the controllers do a lot of heavy lifting in the background such as security, permissions, sorting and filtering.
- View – Templates that render html and CSS for the end-user to see what you want to show them. Laravel uses a php and html template format with no JavaScript interactivity by default.
Adding JavaScript to Laravel
As much as I love php, we wanted to provide investors with a modern app-like experience. To do this we needed a modern JavaScript framework such as React (from Facebook), Angular (from Google) or Vue (an open-source JS framework). I chose Vue because it’s popular in the Laravel community, not owned by a giant tech company and is the fastest to learn for a non JS developer like me.
There are several approaches to getting front-end JavaScript frameworks to work with back-end applications like Laravel:
Approach | Advantages | Disadvantages |
Single Page Application with API – The traditional approach has been to build the JS front-end as a single page application and feed the data through to the app using a private API. | By keeping things separate, it’s easy to focus on one thing at a time. Back-end developers can work on the back-end code and front-end developers can focus on the front-end code. | My experience with SPAs was that I wasted a lot of time keeping the API and the front-end talking to each other. Frustrating points included login tokens and data-table filtering. In a small in-house tech team there’s no such thing as separate back-end and front-end teams, we’re all full-stack by necessity. |
Livewire – This approach allows you to use little bits of JavaScript inside your normal php templates. | It’s cool to have php everywhere and JavaScript only where you most need it. Like a modern framework approach to JQuery. | The disadvantage of Livewire is that it’s still mainly using php not JavaScript, so it doesn’t have the app-like feel of an SPA. It’s also not full-on best-practice JavaScript using Vue or React so you miss out on the Vue and React templates and resources. |
Inertia – This is a new way to use JavaScript inside the MVC framework of a php app. The Model and Controller are in php and the View is in JavaScript. | Best of both worlds. Inertia lets php do what it does best (databases, models, relationships, security, etc) and JavaScript can do what it does best (render the front-end interface and provide interactivity). | Not technically an SPA. Requires some finesse to make it feel fully app-like and the progress loader is a bit clunky. Inertia puts JS right inside your php monolith so you now have a multi-language code base that might feel weird to some developers. |
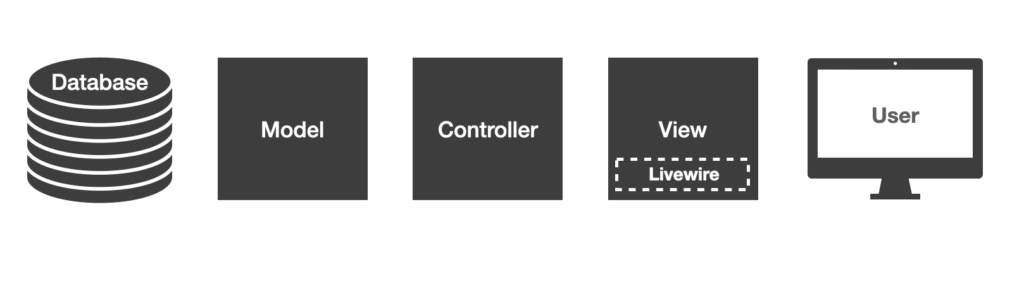
Laravel 8 was released recently and now includes both Livewire and Inertia as options for the default Laravel user interface templates (called Jetstream). This is exciting because it will expose these two new approaches to more developers. But it’s been confusing for a few developers who are used to thinking of JavaScript as a different world from php.
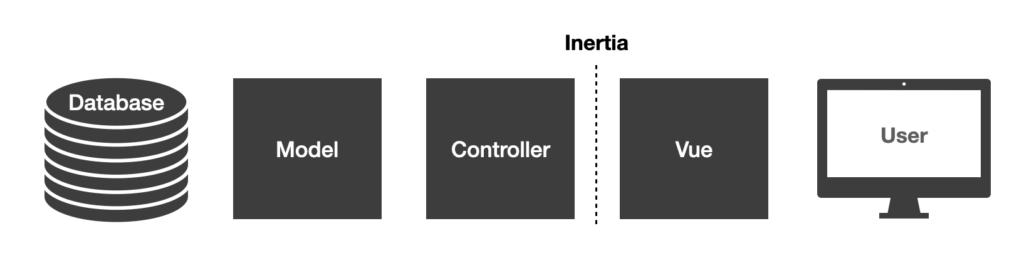
On balance, we opted to use Inertia for the Investor Portal because it kept our code base all in one place and allowed us to move fast and change things quickly based on user feedback. I’ve already had dozens of moments where we wanted to make a change to the way that something is displayed in the portal. It’s a true joy to be able to make a quick change to the Company model, flow it through the Direct Investments controller and have it pop up in the investor portal Vue file all in the same code-base, with easy Laravel debugging and easy feature-testing and front-end testing. By having Vue running inside Laravel I’ve upskilled quickly on JavaScript without having to go all-in on complex JS routing and data manipulation.
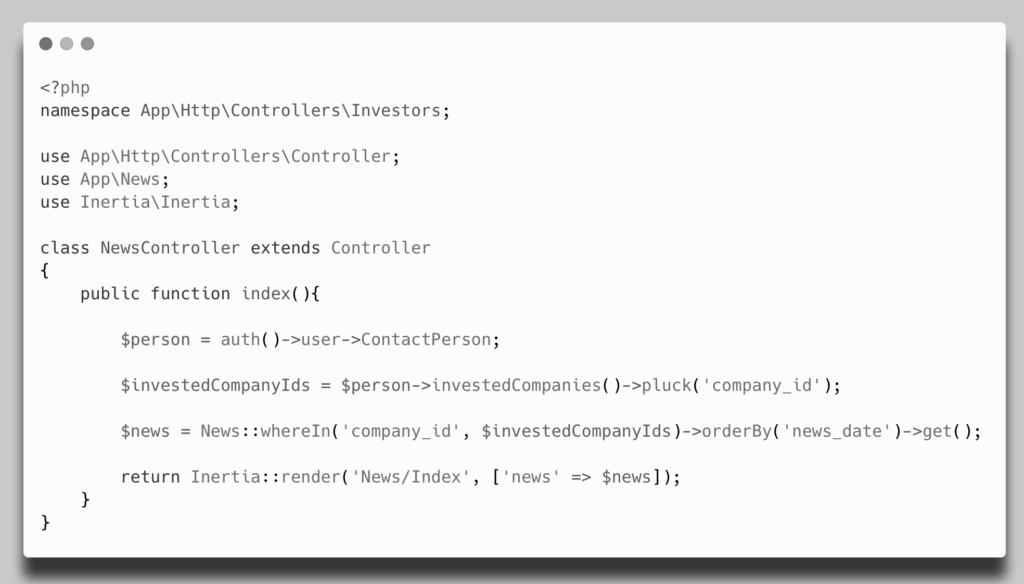
We also went for Tailwind CSS instead of the more established Bootstrap CSS framework. Tailwind is a utility framework approach which means that instead of single giant templates in CSS with little HTML tags you just layer lots of little HTML tags onto an element to make it look the way that you want. It seems messy at first but once you get used to it, it’s life changingly fast. Using Tailwind, Alipne JS, Livewire and Laravel is called the TALL stack and I’m calling Vue, Inertia, Tailwind and Laravel the VITL stack. (Some people prefer the name LIT for this stack but I think Vue is a vital part of what makes this approach so awesome).
Building software in-house is an unusual move for a Venture Capital fund but Icehouse Ventures has a unique approach that combines the scale of the Ice Angels network and the depth of major funds like IVX and Tuhua. Globally, the venture capital industry is being quickly re-shaped by increased investor demands for transparency & reporting and by startup founder demands for faster decision making & streamlined capital raising processes. Technology can be a force multiplier for us and having both php and a modern JavaScript framework like Vue in our toolkit means that we can move faster while still keeping things safe, stable and secure.